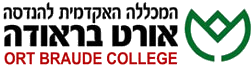
DATA STRUCTURES AND ALGORITHMS 31632
COURSE HOME PAGE
ORT BRAUDE COLLEGE OF ENGINEERING
Electronic and Electrical Engineering Department
Site owner: Samy Zafrany
Course Program
English sylabusHebrew sylabus
Lecture Notes
We will be using materials from the books:- Michael T. Goodrich, Roberto Tamassia, Michael H. Goldwasser, Data Structures and Algorithms in Python. Wiley 2013
- Rance D. Necaise. Data Structures and Algorithms Using Python. Wiley, 2011
- Cormen T.H. et al, Introduction to Algorithm. The MIT press 2001/9, 2nd/3rd ed
- Course Slides Part 01
- Course Slides Part 02
- Course Slides Part 03
- Course Slides Part 04
- Course Slides Part 05 (will not be covered due to insufficient time)
- Course Slides Part 06 - GRAPHS
- Course Slides Part 07 - DFS
- Course Slides Part 08 - BFS
- Course Slides Part 09 - DIGRAPHS
- Course Slides Part 09 - Shortest Path
COURSE PROJECTS
- Before starting each project please read the following Python Coding Style Guides and please make sure to stick to these rules in every line of code that you turn in!
- Every project contains a detailed and precise list of directives on how to do it and how to check it in. Please make sure to read all these direction carefully and act accordingly.
- Make sure you follow exactly the required names to functions, classes, and methods! Any deviation from the suggested name (even with upper or lower case letter difference) can result in your work not being graded, since in many cases your code will be graded by an automatic checker that imports your code and tests it. It is therefore very important that you follow each and every direction in the project text.
-
For this course it is mandatory to understand and follow the following two Style Guides:
- Google Python Style Guide
-
James Madison University Python Coding Conventions (by Nathan Sprague)
These coding style is also based on the PEP8 style guide as described precisely in: PEP8 -- Style Guide for Python Code
- Important Note: viewing Python files (*.py) is best done with Google Chrome browser! In other browsers they might not be recognized as normal text files and thus cannot be viewed (you'll need to download them to disk and then open them with a text editor)
Project 1
You will be redirected to the Python web site. You will find there the project assignment, two different solutions: procedural and object oriented. We have also included the Point/Line classes program that we did in the lab.
Goto
Python Course home page
for materials on the Python programming language.
Project 2
You will be redirected to the Python web site.
The project is NOT to be submitted.
It is for you to gain experience and expertise with the Python programming language,
so please try first to solve it (use the hints), before reading the solutions below.
Goto
Python Course home page
for materials on the Python programming language.
Project 3
Project 4
Project 5
Project 6: Graphs
Final Project: To be submitted at the last week of the semester
EXAMS
-
Materials for final exams, Winter Semester 2014
These are all the slides that are relevant for the final exams. You are allowed to bring them for the final exam, but please do not make any changes or add any hand written notes on them. Note that non-relevant slides (in the original lecture notes) were removed. So this set of slides also defines what you should know for the final examination. - Final Examination 1, Winter 2014
- Solution of Final Examination 1, Winter 2014
- Final Examination 2, Winter 2014
- Solution of Final Examination 2, Winter 2014
Tutorials and Links
- Algorithm Education in Python
- Introduction to Algorithms - MIT Open CourseWare
- Introduction to Algorithms 2 - MIT Open CourseWare
- Project Euler - A great source for Algorithmic Problems
- The Mighty Dictionary
- CS 240: Data Structures and Algorithms, James Madison University, Spring 2013 Professor Nathan Sprague
- 15 Sorting Algorithms in 6 Minutes (YouTube)
- Tim Sort
SOFTWARE
-
C/C++ Programming Languages: Daniel Geva Web Sites
This is the official 31616 course web site of the C/C++ programming languages (owned by Daniel Geva). Most of the Python Data Structures and algorithms are implemented in the C programiing language, and therefore we will occasinally see C programs that explain how a specific data structure was implemented, or how a specific algorithm was made to run fast by writing it in C. So if you haven't practicing C code lately, please visit this course and refresh your C skills soon! -
Python Programming Environment
We will be using the Python Programming Language for many course assignments and projects
since it is ideal for expressing algorithmic ideas just like a pseudo language and at the same time it also runs the algorithms (unlike a pseudo language which is descriptive but cannot run). The link above contains tutorials, books, and software downloads that we need for our course. Please visit this page soon to get acquainted with the Python programming language: we expect the students to be able to learn most of what we need from this language by themselves, as we will not have time to cover all the aspects of the language.
Click here for software installation
Kernighan's Law: Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.
The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!